[SAP ABAP] Data Dictionary
The Data Dictionary is a set of information describing the objects of a database and the relationship between its elements.
The Data Dictionary includes a domain, Types (StRUCTURES, DATA TYPE, and TABLE TYPE), tables, views, search helps, and lock objects.
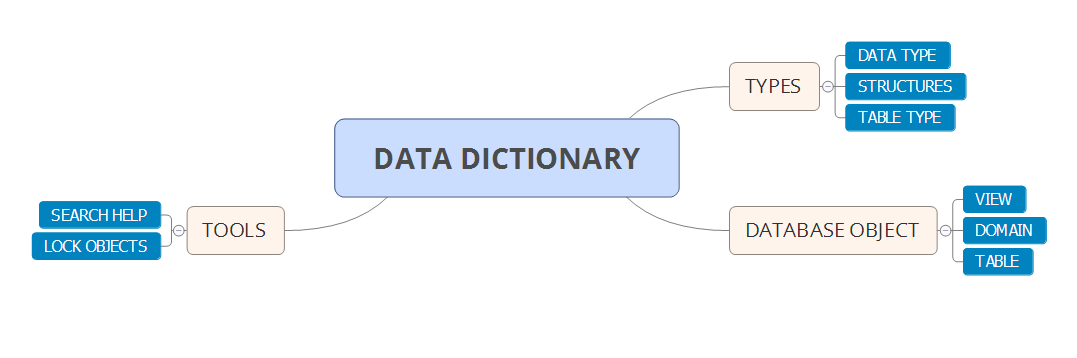
To create an object for the Data Dictionary, we need to use the SE11 transaction. The transaction code is usually only a few characters long and guides you directly to the screen of the task that the user wants to perform. This screen is shown in the following screenshot:
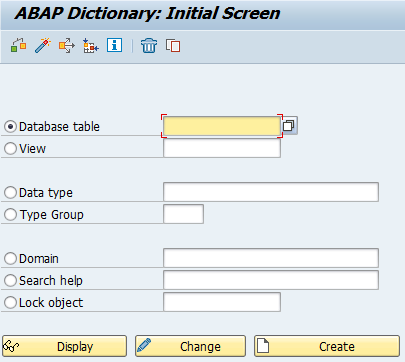
A domain is an object that describes various technical attributes, such as the data type length of a table field. A domain is assigned to a data element. One domain can be assigned to many data elements.
Creating a domain is very easy and quick. We don't need specialized knowledge. The following is the typical procedure for creating the data element:
- Go to
Transaction SE11
, select the radio button forDomain
, and enter the name you wish to give it, preferably something you will remember when you see it. Then, click onCreate.
It is important that the names of the domains follow the convention ofZ
(orY
) prefixes:
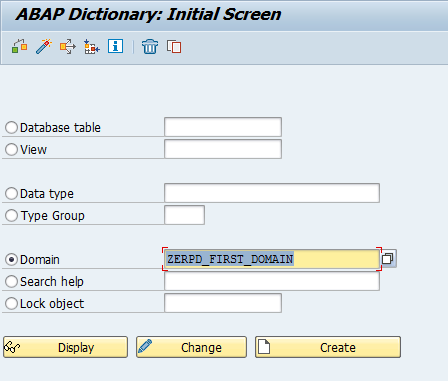
- The
Definition
tab defines the technical data. The user enters the description of the data. In this step, we must select the data type (what kind of data this is), as well as the number of characters. Fortunately, the SAP environment is very friendly. If we forget to fill in a field that's mandatory, the system will not allow you to save the changes. We have the option to enter a decimal place if we choose a numeric data type. Conversion is the procedure that SAP uses to normalize the data held in the various tables, but it is not obligatory. There are two methods in a conversion routine. The first adjusts the data to the variable, while the other changes the data so that it's in a more readable output form.
In summary, this is a way we can adjust the values to variables. The
Lower Case
checkbox allows you to store lowercase characters (as stated by its label). By default (when it is not checked), the values are stored in uppercase. The following screenshot explains what fields you need to fill in to create a domain: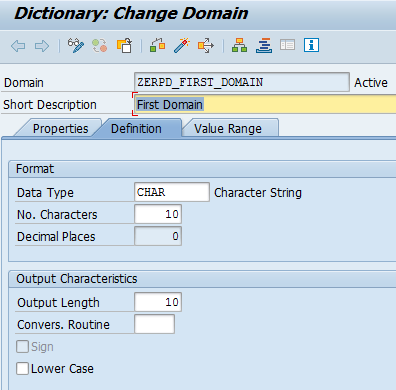
- All the mandatory fields have been filled in so we can save our domain. In the SAP system, if we want to use an object, we must activate it. Activation is the process by which execution causes the object to be made available to the runtime environment. This means that it can be used or started.
Value Range
is a very useful option. Often, we need to use specific data. We can define a single value or value table for the domain.Value Table
is one of the properties of a semantic domain and contains the default values of the field control table. Only the values contained in this table can be used. The following screenshot explains how to define a value in a domain:
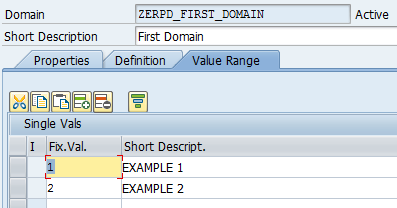
DATA: lt_value_domain TYPE TABLE OF dd07v.
CALL FUNCTION 'DD_DOMVALUES_GET'
EXPORTING
text = 'X'
domname = 'ZERPD_FIRST_DOMAIN'
TABLES
dd07v_tab = lt_value_domain.
The result of the preceding program is a table with values:
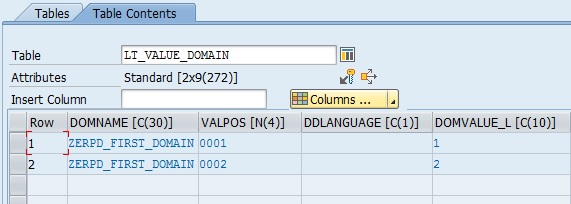
Now let's move on to the next section.
Data elements are objects that describe the unitary fields in the Data Dictionary. It should be emphasized that the domain is used to define technical parameters, while the data element is more function-oriented (because it has labels explaining its purpose). This object is used to define the type of the table field, as well as the structure of the component.
Follow these steps to create a data element:
- Go to
Transaction SE11
, select the radio button for theData Type
element, and enter a name for it. Then, click onCreate
:
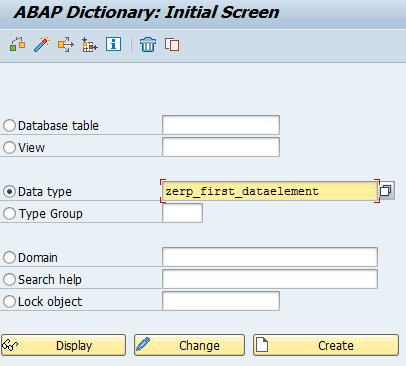
- Choose the
Data element
checkbox and press Enter:
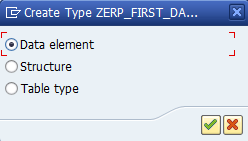
- Enter a short description and assign the element with the type. The user can assign one of the following types to the data:
- Domain
- Elementary type
- Reference type
The following screenshot explains the data element creation screen:
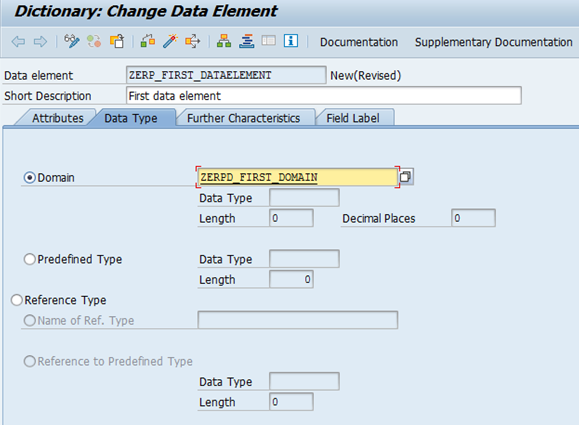
- Enter the fields for
Short
text,Medium
text,Long
text, andHeading
in theField Label
tab, as shown in the following screenshot. Then, save and activate your changes:
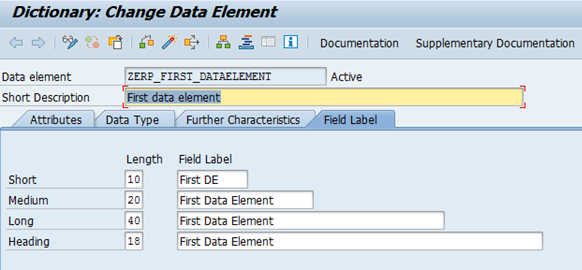
The structure is a data object that consists of components or fields. This data object can be created globally and locally. The user can create a global structure using the SE11 transaction. The creation of a local structure can be implemented in two ways. The first way is by implementing the code in the method:
TYPES: BEGIN OF tt_type,
first_data TYPE string,
second_data TYPE i,
third_data TYPE c,
END OF tt_type.
This solution has the limitation that it is only implemented in one method. If we want to use a structure in another class, we must create types in the class editor:
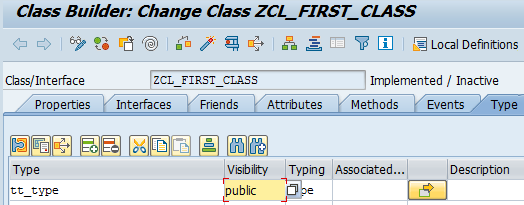
When we enter
Direct Type Entry
, we develop the preceding code.
A structure can be used in classes, report programs, interfaces, and module pools. If we create a table, we use a structure to determine what fields will be used. A structure may have only a single record at runtime.
The creation of a structure in the SAP system can also be performed using SE11 Transaction.
In the
Data type
field, the name of the created structure is entered and the Create
button must be pressed. The window of this transaction with the structure name entered is as follows: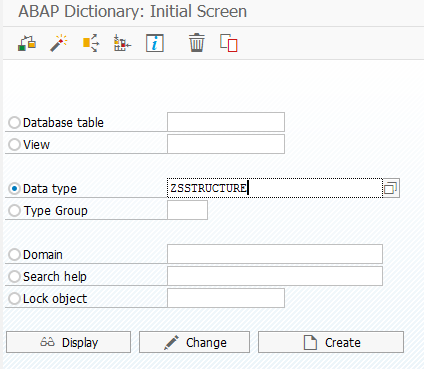
The system asks what type of data the user wants to create. The user should choose
Structure
. After entering the creation screen, enter a short description of the structure being created. In the window that appears, you can enter any variable names and specify their type. There are two ways of declaring variables in the structure. The first option is to use existing data types. The user can also use predefined data types. For example, the completed fields of the structure are shown in the following screenshot: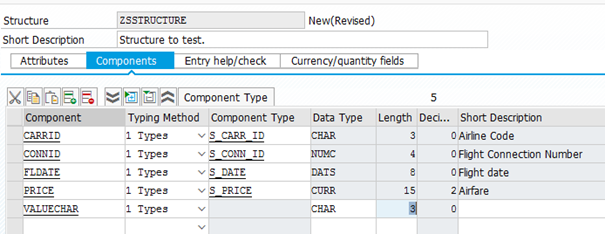
In the
Attributes
section, information about the structure creator, last change time, and the package is available. This information is useful when the structure needs to be expanded and there is a need to consult its creator. To use the created structure, the creator should save and activate it. A structure that's created in this way can be used in any program in the SAP system.
One of the most important elements of the Data Dictionary is its ability to help you implement search helps. It allows you to find all the required values. Of course, if we need to narrow down our search, we can do that. Creating a search help in SAP is very simple and is used often.
There are two types of search helps in the SAP system:
- A collective search help
- An elementary search help
A collective search help is a combination of several elementary searches. This search help object specifies a series of search process paths for the object. This category has an interface that carries values because the results are transferred between the basic search help and the input template.
An elementary search help is used to display the possible entries of values into the field. This helps in completing the fields as we can select values from the list.
In order to create a search help, the user should select SE11 transactions and select the appropriate checkbox. The next step is to enter a name and press the
Create
button. In the next window, the system will ask what category the user wants to choose. For the example shown, Elementary srch hlp
was selected. After accepting this type of category, the system will display the creation screen, as follows: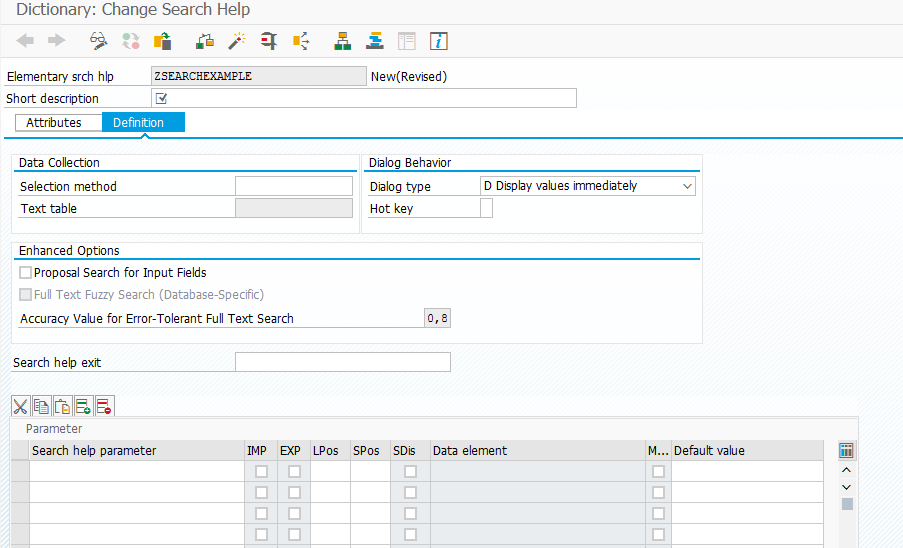
Just like many other objects in the SAP system, a short description is obligatory. In the
Selection method
field, the user can enter tables that values will be collected from. The type dialog is used to specify the appearance of the limiting dialog box. To use the search help tool, the system requires the selection of the parameters that will be used. The user can do this in the parameter section. In the parameter section, it is possible to fill in the following fields:- Import (
IMP
): Determines whether the parameter is imported - Export (
EXP
): Determines whether the parameter is exported LPos
: Entering the value determines where the field will appear in the selection listSPos
: Entering the value determines where the field will appear in the restrictive dialog box
An example screen with completed data is as follows:
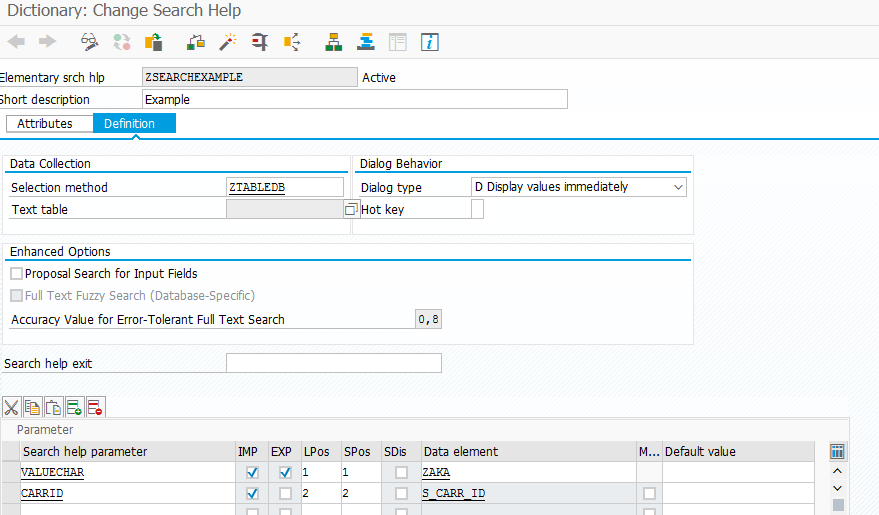
After creating, saving, and activating, it is possible to test the search help tool that was created. In this example, the search help is as follows:
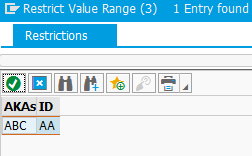
As we can see, the search help works without any problems.
The table type is one of the elements of the ABAP dictionary in the SAP system. Its main purpose is to defend the internal table and the sourcing of structures. Like the other elements, it is created in Transaction SE11. A table type created by this transaction is available globally. This means that, once created, an object can be used in many programs, not depending on the place. Changes in these objects must be thought out because they can affect many processes and cause their incorrect operation. The second way to create a type table is to create it locally. It is then included in the program's code and is only available in this area.
During the creation of a types table in a SE11 transaction, the user can choose one of the following types of data access:
- A standard table
- A sorted table
- A hashed table
- An index table
- Not specified
Comments
Post a Comment